Introduction:
Table of Contents
Flutter, Google’s UI toolkit, is widely appreciated for its ability to create natively compiled applications for mobile, web, and desktop from a single codebase. One of the reasons for its popularity is the vast array of packages available that extend its functionality and ease of development. In this article, we’ll explore the top 5 important packages in Flutter, providing examples to help you understand their usage and benefits.
1. Provider:
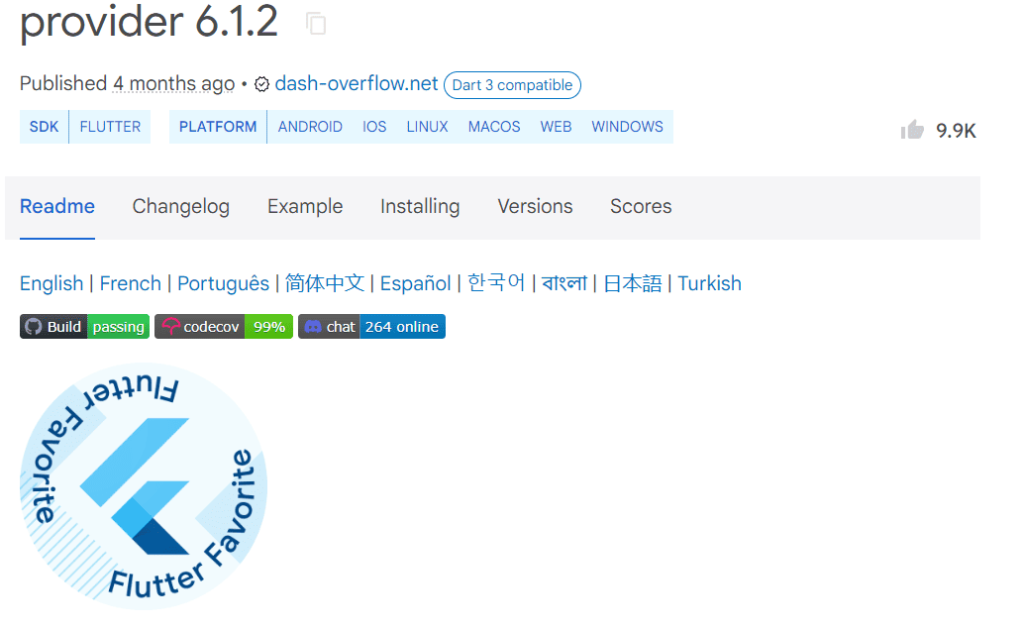
Overview:
Provider is a highly recommended package for state management in Flutter. It offers an efficient way to manage and share state across your app, making it easier to build reactive applications. Unlike other state management solutions, Provider is built with simplicity and performance in mind, leveraging the InheritedWidget in Flutter to propagate changes throughout the widget tree efficiently.
Features:
- Easy to use and integrate.
- Optimizes performance by minimizing rebuilds.
- Supports dependency injection.
Example:
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
}
class Counter with ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Provider Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('You have pushed the button this many times:'),
Consumer<Counter>(
builder: (context, counter, child) {
return Text(
'${counter.count}',
style: Theme.of(context).textTheme.headline4,
);
},
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: () => Provider.of<Counter>(context, listen: false).increment(),
tooltip: 'Increment',
child: Icon(Icons.add),
),
),
);
}
}
2. HTTP:
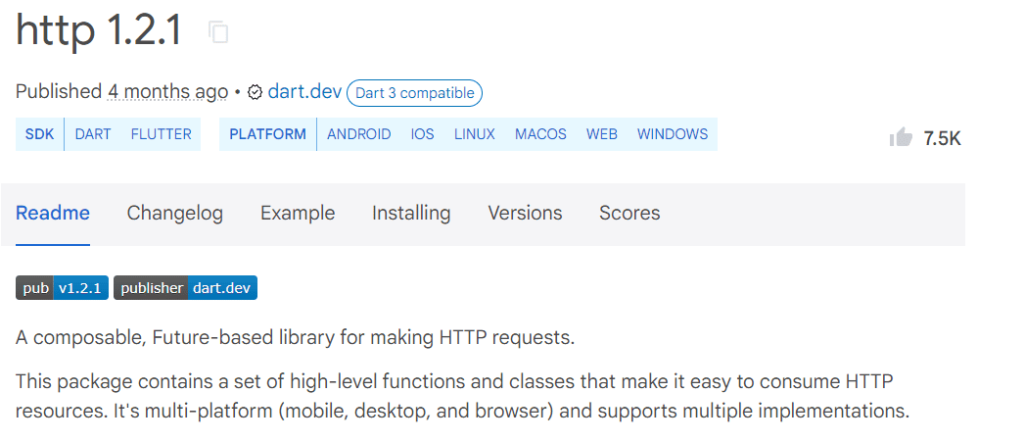
Overview:
The http
package is indispensable for making network requests in Flutter. It simplifies the process of fetching data from APIs, posting data to servers, and handling various HTTP methods. This package is particularly useful when your app needs to interact with web services, RESTful APIs, or perform any network operations.
Features:
- Simple API for making HTTP requests.
- Supports all standard HTTP methods (GET, POST, PUT, DELETE, etc.).
- Handles asynchronous operations with ease.
Example:
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
import 'dart:convert';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('HTTP Example')),
body: Center(child: DataFetcher()),
),
);
}
}
class DataFetcher extends StatefulWidget {
@override
_DataFetcherState createState() => _DataFetcherState();
}
class _DataFetcherState extends State<DataFetcher> {
String data = "Fetching data...";
@override
void initState() {
super.initState();
fetchData();
}
fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts/1'));
if (response.statusCode == 200) {
setState(() {
data = json.decode(response.body)['title'];
});
} else {
setState(() {
data = "Failed to fetch data";
});
}
}
@override
Widget build(BuildContext context) {
return Text(data);
}
}
3. Flutter_bloc:
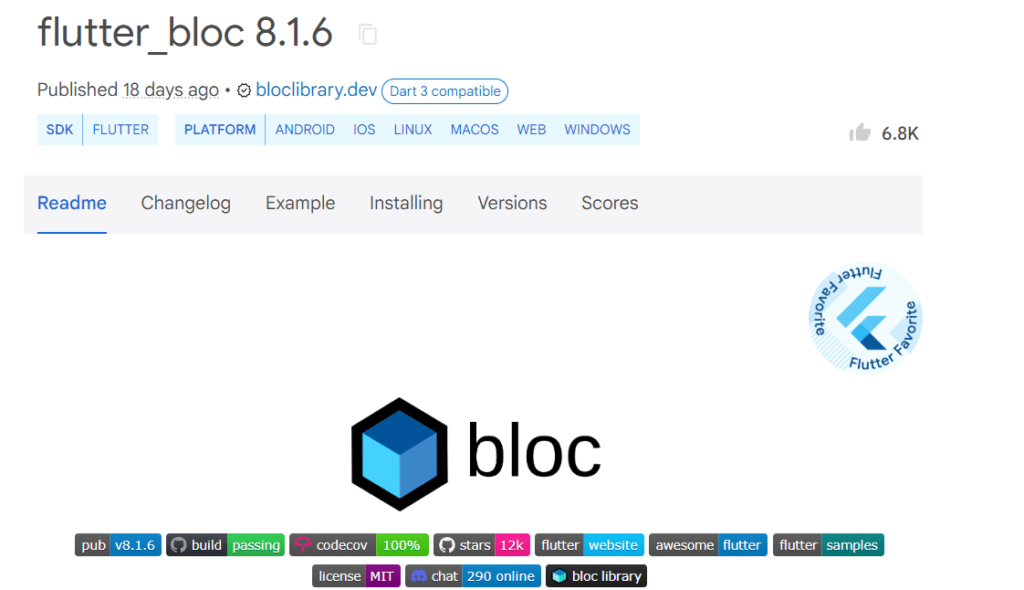
Overview:
flutter_bloc
is a powerful state management package that implements the BLoC (Business Logic Component) pattern. It helps in separating business logic from UI, making the code more modular and testable. This package is ideal for managing complex state and ensuring that the business logic is decoupled from the presentation layer.
Features:
- Promotes a clear separation of concerns.
- Simplifies state management in complex applications.
- Integrates well with other Flutter packages.
Example:
import 'package:flutter/material.dart';
import 'package:flutter_bloc/flutter_bloc.dart';
// Define Events
abstract class CounterEvent {}
class Increment extends CounterEvent {}
// Define States
class CounterState {
final int count;
CounterState(this.count);
}
// Define Bloc
class CounterBloc extends Bloc<CounterEvent, CounterState> {
CounterBloc() : super(CounterState(0));
@override
Stream<CounterState> mapEventToState(CounterEvent event) async* {
if (event is Increment) {
yield CounterState(state.count + 1);
}
}
}
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: BlocProvider(
create: (context) => CounterBloc(),
child: CounterPage(),
),
);
}
}
class CounterPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Bloc Example')),
body: Center(
child: BlocBuilder<CounterBloc, CounterState>(
builder: (context, state) {
return Text('Counter: ${state.count}');
},
),
),
floatingActionButton: FloatingActionButton(
onPressed: () => context.read<CounterBloc>().add(Increment()),
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
4. Shared Preferences:
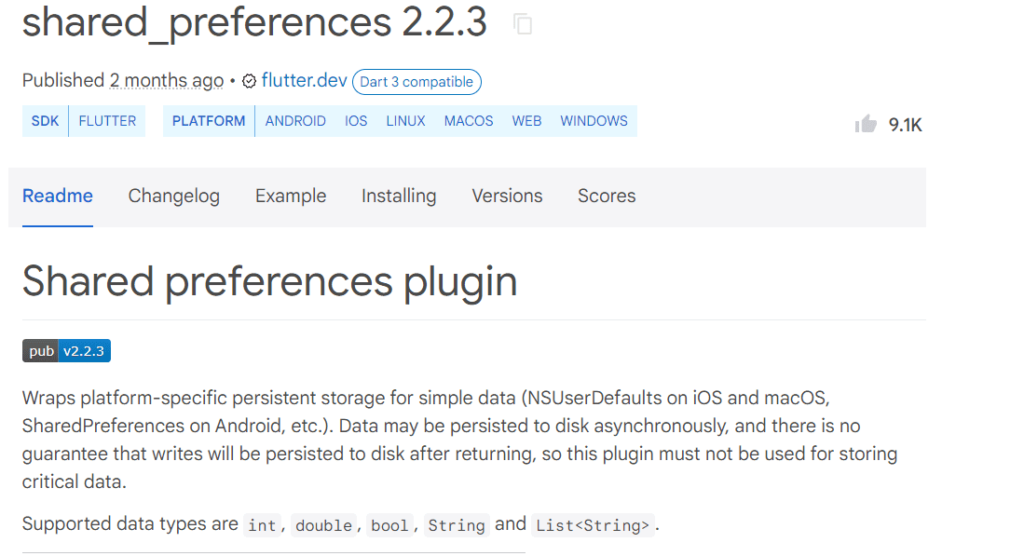
Overview:
The shared_preferences
package provides a simple way to store and retrieve small amounts of data, such as user settings or preferences, in a key-value format. It is ideal for storing non-sensitive data that needs to persist across app launches.
Features:
- Easy-to-use API for persistent storage.
- Stores data in a key-value format.
- Suitable for storing user preferences and settings.
Example:
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: SharedPreferencesExample(),
);
}
}
class SharedPreferencesExample extends StatefulWidget {
@override
_SharedPreferencesExampleState createState() => _SharedPreferencesExampleState();
}
class _SharedPreferencesExampleState extends State<SharedPreferencesExample> {
int _counter = 0;
@override
void initState() {
super.initState();
_loadCounter();
}
// Load counter value from shared preferences
_loadCounter() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
setState(() {
_counter = (prefs.getInt('counter') ?? 0);
});
}
// Increment counter and save value to shared preferences
_incrementCounter() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
setState(() {
_counter++;
prefs.setInt('counter', _counter);
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Shared Preferences Example'),
),
body: Center(
child: Text(
'Counter: $_counter',
style: TextStyle(fontSize: 24),
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
5. Firebase:
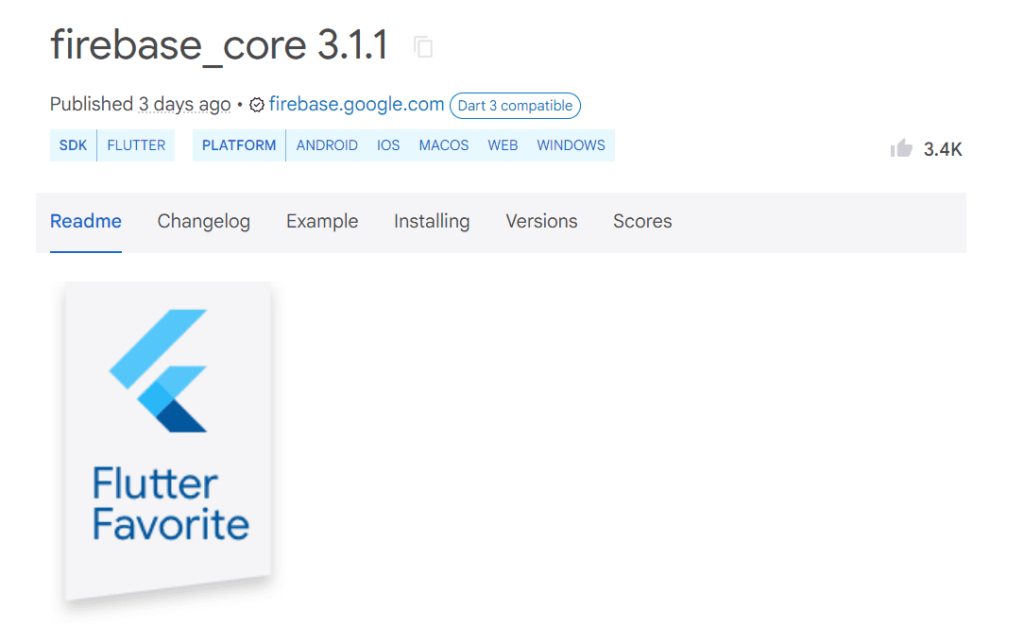
Overview:
Firebase is a comprehensive platform provided by Google for mobile and web application development. In Flutter, Firebase services are available through various packages, such as firebase_core
for Firebase initialization and firebase_auth
for authentication. Firebase offers a range of backend services, including real-time databases, cloud storage, authentication, analytics, and more.
Features:
- A comprehensive suite of development tools.
- Easy integration with Flutter.
- Provides authentication, database, storage, and analytics.
Example:
import 'package:flutter/material.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:firebase_auth/firebase_auth.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: AuthExample(),
);
}
}
class AuthExample extends StatefulWidget {
@override
_AuthExampleState createState() => _AuthExampleState();
}
class _AuthExampleState extends State<AuthExample> {
final FirebaseAuth _auth = FirebaseAuth.instance;
User? _user;
@override
void initState() {
super.initState();
_auth.authStateChanges().listen((User? user) {
setState(() {
_user = user;
});
});
}
Future<void> _signInAnonymously() async {
try {
await _auth.signInAnonymously();
} catch (e) {
print(e);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Firebase Auth Example'),
),
body: Center(
child: _user == null
? ElevatedButton(
onPressed: _signInAnonymously,
child: Text('Sign in Anonymously'),
)
: Text('Signed in as ${_user!.uid}'),
),
);
}
}
Conclusion:
These top 5 Flutter packages—Provider, HTTP, flutter_bloc, Shared Preferences, and Firebase—are essential tools for any Flutter developer. They simplify state management, networking, storage, and integration with powerful backend services. By mastering these packages, you can create robust, efficient, and scalable Flutter applications.
Staying updated with the latest versions and best practices for these packages will help you maintain and improve your app’s performance and reliability.